Adding custom Javascript to the quiz
For developers experienced with JavaScript, Lantern Product Quiz Builder offers a powerful suite of events and functions that can be used to enhance quiz interactivity and data handling. This guide explores these capabilities, detailing each event and function.
Where/how to add custom Javascript code
Lantern has a custom Tracking Code section under Settings where you can input any JavaScript code. To start, click Edit Quiz, and go to Settings.
We have 2 separate sections- one for code that should be inserted in the head of the page and one for code that should be added to the body of the page.
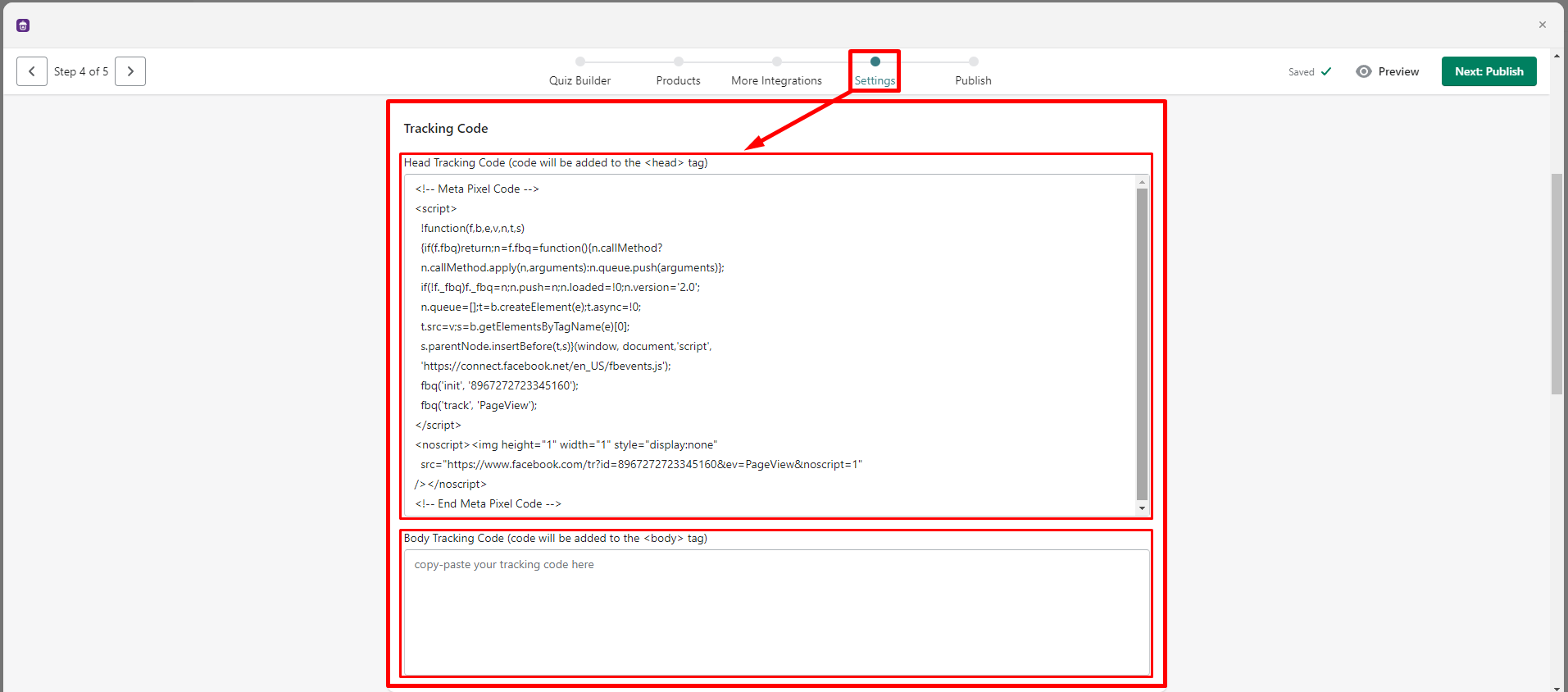
Please note: For optimal performance and reliability of your JavaScript customizations in the Lantern Product Quiz Builder, it is recommended to place your JavaScript code in the body section of the Tracking code input box, rather than in the header.
JavaScript Events in Lantern
1. lantern:reach_results_page
- Triggered: When the results page is reached but before any results are displayed.
- Use case: Ideal for redirections or pre-result manipulations.
window.addEventListener("lantern:reach_results_page", () => {
window.location.href = "https://alternative-website-for-results.com";
});
2. lantern:display_results
- Triggered: When the quiz results are ready to be displayed.
- Properties:
- email : Email address of the user (if provided).
- first_name : First name of the user (if provided).
- marketing_consent : Whether the user has consented to marketing.
- results : Array of product recommendations.
- content_block_results : Results from content blocks.
- answers : Answers given by the user (only answers with a name are available; assign a Property ID to each question in the quiz builder).
window.addEventListener("lantern:display_results", (e) => {
const { results, content_block_results, answers, email, first_name, marketing_consent } = e.detail;
console.log({ results, content_block_results, answers, email, first_name, marketing_consent});
console.log(`${first_name} provided their email address: ${email} and has${marketing_consent === "YES" ? "" : " not"} agreed to receive marketing emails.`);
for(const result of results) {
console.log(`Recommended product: ${result.product.title}`);
}
});
This code will display the available properties in your browser's console, allowing you to inspect the quiz data in real-time.
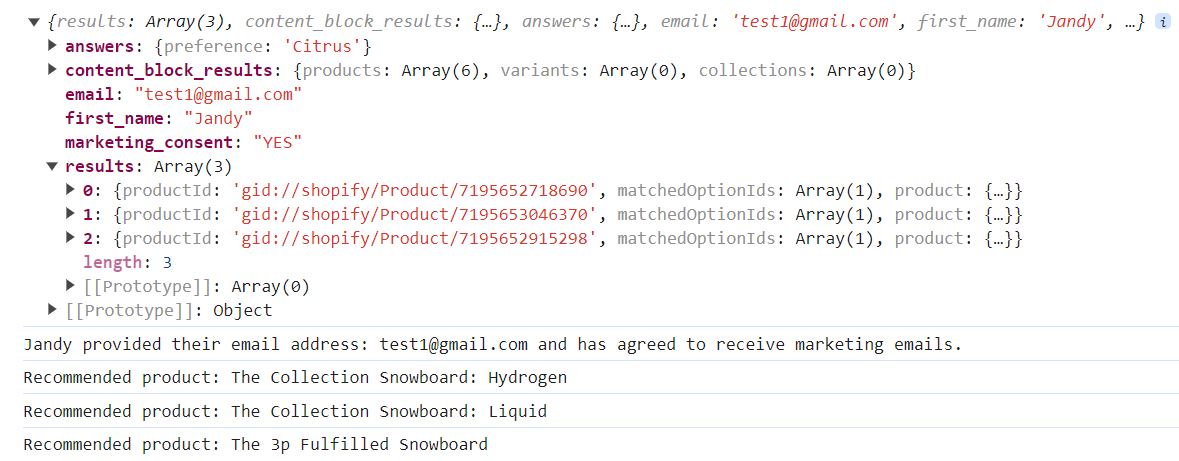
3. lantern:display_question
- Triggered: When any quiz page is displayed.
- Properties:
- id : Unique identifier of the page.
- title : Title of the page.
- description : Description of the page.
- type : Type of page (e.g., question, email capture).
- options : Available options for the question.
- isRequired : Whether the response to the page is required.

4. lantern:submit_answer
- Triggered: When an answer is submitted.
- Properties:
- id, title, description, type, options, isRequired : Identical to display_question.
- answers : Array of submitted answers.
- Additional properties on email capture pages:
- email : Email address of the user.
- first_name : First name of the user.
- marketing_consent : Marketing consent status.
window.addEventListener("lantern:submit_answer", (e) => {
const { answers, email, first_name, marketing_consent } = e.detail;
console.log({ answers, email, first_name, marketing_consent });
});
This code will show the available properties for the answer(s) in your browser’s console.

JavaScript Functions in Lantern
1. window.lantern.addAllToCart()
- Asynchronously adds all recommended products to the shopping cart. This function is particularly useful for dynamic content blocks with product recommendations. Note that products requiring variant selection cannot be added automatically.
async function handleAddAllToCart() {
await window.lantern.addAllToCart();
console.log("All products added to cart.");
}
2. window.lantern.goToCart()
- Opens the shopping cart page in a new tab. This function is used immediately after addAllToCart to direct users to review their cart, enhancing the shopping experience.
function openCart() {
window.lantern.goToCart();
}
3. window.lantern.restartQuiz()
- Resets the quiz to its beginning, enabling users to retake the quiz without reloading the page. This function is especially valuable for developers to integrate within their custom JavaScript implementations, ensuring users can start over smoothly.
function restartQuiz() {
window.lantern.restartQuiz();
}
4. window.lantern.closeQuiz()
- Closes the quiz interface. This function mimics the action of the quiz's close button and is useful for developers who want to programmatically end the quiz experience from custom scripts.
function closeQuiz() {
window.lantern.closeQuiz();
}
For any questions or enquiries please contact our support team.